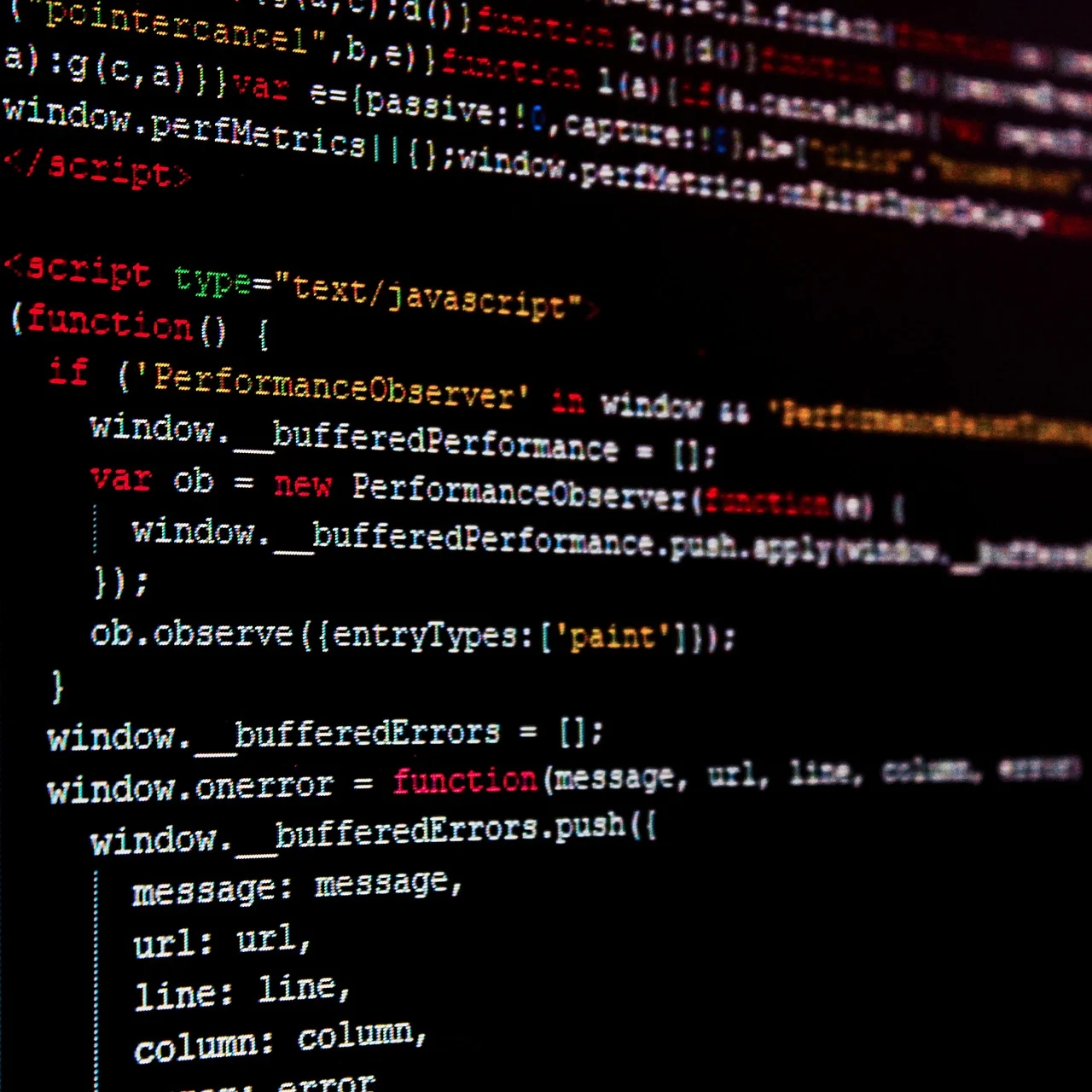
Abstract objects can have multiple implementations. Fortran does not offer a direct solution to declare them as an array. However, it is still possible to handle it. Check below how to do it:
Abstract type:
type, abstract :: shape
...
end type
Several implementations:
type, extends(shape) :: circle
...
end type circle
and
type, extends(shape) :: square
...
end type
Declare a dynamic array of concrete objects:
class(square), allocatable :: tiles(:)
Declare a dynamic array of the abstract type shapes:
First, declare a wrapper of the abstract class:
type :: shapeWrapper
type(shape), allocatable :: sh
end type
Then, the array can be normally operated:
type(shapeWrapper), allocatable :: shapes(:)
Finally, Client code:
allocate(shapes(3))
allocate(square:: shapes(1) % sh)
allocate(circle:: shapes(2) % sh)
allocate(square:: shapes(3) % sh)
Further customizations can be applied to the wrapper class to make it more transparent. As an example, overload the (==) assignment, as well as the (=) operator…
That would help the developer deal with less code complexity with the shown workaround.